Promise 的基本工作原理
实现思路
首先创建一个 Promise 类,包含状态、值和回调数组,实例化时接收一个回调函数
创建一个方法 then,接受回调函数,判断状态,执行回调或存入回调数组
创建一个方法 resolve,接受值,判断状态,执行回调或存入回调数组
创建一个方法 ces,返回一个 Promise 对象
用到的知识点
bind 方法:改变方法的上下文
callback 函数:回调函数
代码实现
class MyPromise {
constructor(callback) {
this.state = "pending"; // 初始状态
this.value = undefined; // 初始值
this.onCallback = []; // 回调数组
callback(this.resolve.bind(this)); // 传入resolve方法
}
resolve(value) {
if (this.state === "pending") {
this.value = value;
this.state = "fulfilled";
this.onCallback.forEach((fn) => fn());
}
}
then(callback) {
if (this.state === "fulfilled") {
callback(this.value); // 如果走到这里完成了,则说明mypromise里面运行时的同步任务
} else {
this.onCallback.push(() => callback(this.value)); // 否则存入回调数组
}
}
}
const ces = () => {
console.log("直接输出");
return new MyPromise((resolve) => {
setTimeout(() => {
console.log("3秒后输出");
resolve(1);
}, 3000);
});
};
ces().then((res) => {
console.log(res);
});
执行流程
执行 ces()时,立即打印"直接输出"
创建 Promise 实例,设置 3 秒定时器
执行.then()时,因为状态还是"pending",所以回调被存入 onCallback 数组
3 秒后,定时器触发:
打印"3 秒后输出"
执行 resolve(1)
状态变为"fulfilled"
触发存储的回调,打印 1
关键步骤
ces()是同步执行到 then 的,ces 里面有异步方法则进入异步队列,继续执行 then 方法。
说明图
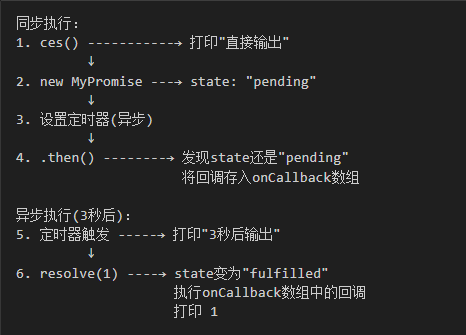